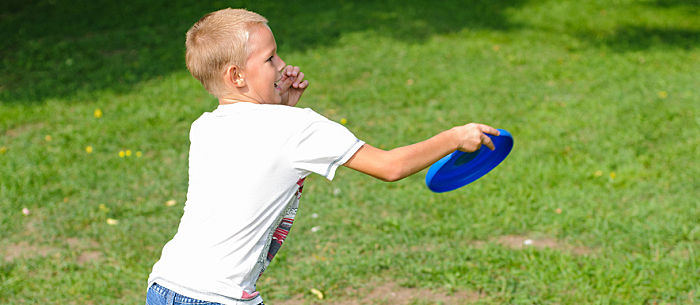
For writing your REST API automation test cases there are many tools available like Runscope, Fit Selenium, PyRestTest, REST-assured and many such more. Before selecting the right tool for REST automation some important aspects to consider are:
Architecture
The framework components need to be loosely coupled to each other and there should be a generic way to invoke test cases and retrieving results. The framework design should be such that, any changes in REST API should least impact the automation scripts.
Data structures
The framework should support all the data formats supported by REST APIs like XML, JSON, Multi-Part data, plain text etc.
Assertions
Most of your REST API testing will revolve around validations. A good framework should provide out of the box support for such validations/comparisons. Some validation examples: verifying API response code, response headers, verifying the response body.
Reporting
A testing framework is of no use if it lacks the ability to generate reports. Reports give you a clear visibility of your time and efforts invested in automation. There should be reporting mechanism implemented that should define the output in user readable format. This kind of report will help us to design the failures/success rate in REST API automation.
After considering all the above points, I decided to stick with Frisby. Frisby is a REST API testing framework built on node.js and Jasmine that makes testing API endpoints easy, fast, and fun. Its as simple as tossing a frisby.
Jasmine is a behavior-driven development framework for testing JavaScript code. It does not depend on any other JavaScript frameworks and most importantly, it does not require a DOM. It has a clean, obvious syntax so that you can easily automate your tests.
Sample GET request in Frisby:
var frisby = require('frisby');
var URL = 'https://cubicrace.com/widgets/middleware';
frisby.globalSetup({ // globalSetup is for ALL requests
request: {
'Accept': 'application/json',
'Content-Type': 'application/json',
'Authorization': 'Basic YWRtaW46YWRtaW4='
},
timeout: (60 * 1000)
});
frisby.create("Positive_GET_MiddlewareMonitor_Widget_Status")
.get(URL)
.afterJSON(function(json) {
expect(json.ldapStatus).toMatch("started");
expect(json.dbStatus).toMatch("started");
})
.expectStatus(200)
.expectHeaderContains('content-type', 'application/json')
.toss();
Sample POST request in Frisby:
var frisby = require('frisby');
var URL = 'https://cubicrace.com/widgets/middleware';
frisby.globalSetup({ // globalSetup is for ALL requests
request: {
'Accept': 'application/json',
'Content-Type': 'application/json',
'Authorization': 'Basic YWRtaW46YWRtaW4='
},
});
var postData = {
"propertyFile":"blog.properties",
"propertyName":"ui.cubicrace",
"propertyValue":"passive"
};
frisby.create("Positive_Create_Property")
.post(URL, postData, {json: true})
.afterJSON(function(json) {
expect(json.result).toMatch("property_updated");
})
.expectStatus(200)
.toss();
Frisby is exceptionally good at checking the values in a JSON response against
the specified values (see the "expect" statements in above examples). Frisby also allows you to perform type checking, useful for making sure
you’re getting the types that really should be there, like a number
rather than a string representation of a number.A list of expectations that can be chained:
expectHeader
expectHeaderContains
expectJSON
expectJSONTypes
expectJSONLength
expectBodyContains
expectHeaderContains
expectJSON
expectJSONTypes
expectJSONLength
expectBodyContains
Since Frisby is JavaScript, you can write some additional JS functions to make your automation more strict and powerful. Frisby can be used with a "Continuous Integration" system or run with each build to perform some of your Build Verification Tests (BVT)
Link to documentation: Frisby.js API Documentation
Note: Frisby is built on top of the Jasmine BDD (Behaviour-Driven Development) framework, and uses the jasmine-node test runner to run spec tests.
Some of the build-in validation statements:
var a = true;
expect(a).toBe(true);
var b = false;
expect(b).not.toBe(true);
//Works for simple literals and variables
var a = 12;
expect(a).toEqual(12);
//The 'toMatch' matcher is for regular expressions or strings
var message = "foo bar baz";
expect(message).toMatch(/bar/);
expect(message).toMatch("bar");
expect(message).not.toMatch(/quux/);
//The 'toBeDefined' matcher compares against `undefined`
var a = { foo: "foo" };
expect(a.foo).toBeDefined();
expect(a.bar).not.toBeDefined();
//The 'toBeLessThan' matcher is for mathematical comparisons
var a = 12.5,
b = 2.5;
expect(b).toBeLessThan(a);
expect(a).not.toBeLessThan(b);
expect(a).toBeGreaterThan(b);
expect(b).not.toBeGreaterThan(a);
For a full list of supported validation statements see the Jasmine Api Documentation.Next Reading: How to Install Frisby on Linux